Egemen Koku
I love algorithms - especially when they love me back ^-^
Oh and also, video games are pretty cool.
-
Tools Programming
-
Used SFML-based UI library called SFGUI to build a level editing tool from scratch to edit the game objects and save / load levels to iterate faster.
-
-
Engine Programming
-
The engine is using a hybrid component-based and object oriented design as its architecture. Component-based design is used to define game objects and add or remove functionality from them. The object oriented design is used for all the back-end systems and fundamental classes or interfaces.
-
Redesigned the existing game object management from our old engines into a centralized and scalable system to improve performance and development speed.
-
The engine is using JSON format for all configuration and level files. I've written a JSON parser using rapidjson, a light-weight JSON utility library to change level data as well as use different files for different configurations.
-
Written object serialization from scratch in a scalable way in order to make adding / subtracting entities and/or components swiftly.
-
Save / Load file functionality for JSON format. This is used for level editing and configuration as well as game save data and object serialization.
-
Developed 3D Audio library for music and sound effects.
-
-
Graphics Programming
-
Implemented a GPU-based particle system that handles all particle creation, management and movement in shader-code. My implementation was using a double-buffered version of the ogldev article for a better performance.
-
Unlit is a 2.5D platformer game we did as a four people team in a semester. For this game, we made a game engine in C++ from scratch and developed the game on it. I'm quite proud of it for the entire game is built in about 3 months including the engine development.
The game is about a small girl falling into a cave and then trying to find the exit with the help of a light spirit she met in the cave. It has four different levels and simple platformer mechanics.
This page will be about my contributions and the technologies used in the project. For the other awesome people worked on the game:
-
Lisa Sturm - Gameplay Programmer
We dubbed our engine as "WhiskyEngine", which was a component-based 3D game engine developed in C++ using various C++ libraries like SFML, GLM, etc... The engine started out as a combination of all our previous engines but soon enough we realized that the functionality of our previous engines weren't good enough for what we wanted to make (we came further than we'd imagined in about a semester. Power of DigiPen I suppose :) ) so we decided to keep the parts that were ok and replace the main code using a different architecture. Since our previous engines were also done in a component-base way, the union was relatively simple.
I was the main engine programmer in our team so I decided on the parts that we need to re-implement and started working on them. We had already decided to use JSON as our resource files (more readable than simple text format) so that was my main task. I found a JSON parser called rapidjson, a light-weight library that is just a few header files, and used that to create an API for parsing our resource data. Developing the API wasn't too difficult; the main time-consuming part was to change all the existing components' initialization logic into using the JSON functionality.
Since I was already working on every single component we already had, I decided to tackle the object serialization as well. Our previous architecture was an archaic one with a sub-optimal performance. I decided to take that as my next task and change the serialization of game objects and how we're handling component creation.
When I was done with changing the skeleton of the engine and adapting existing parts, my other team mates were also done with what was needed to start developing the game. Since we were already using JSON for game objects, we decided to create levels using the JSON files. Boy was that a big mistake! :) It was absolute nightmare in terms of time required to create a level, testing and adjusting it. JSON didn't made it as easy as we thought so we decided to make a very simple level editor, just enough to move game objects around and place them. Since I was mainly done with the engine (and always loved making tools), I worked on this task next.
After I was done with the level editor, I decided to at least make it a proper level editing tool buy adding scaling and rotation information as well as some physics information editing. We didn't think of making a larger level editing tool, and frankly the time was running out, so we decided to keep it as simple as possible but this alone made it very easy for us to edit levels.
Finally, I added the more "game-y" parts to the engine. For the audio, I used SFML's own audio library. For the particles, however, I decided to implement an algorithm I found on nVidia's page - a particle system using GPU for particle movement and creation. It was fairly simple to implement and very fun to test. As a result, we could have hundreds of particles at the same time without hurting the frame rate too much.
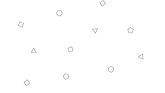
THE ENGINE
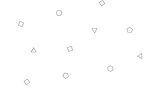
MY ROLE

INTRODUCTION
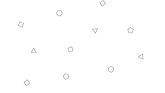
TOOLS
We started without any tool needs in our minds. We believed editing JSON files for game objects would be enough to handle level design and boy were we wrong :)
Our inexperience came to haunt us in the form of realization that we were spending too much time editing a JSON file to get the best platforming experience. As a result, I started looking up on UI libraries that we could use with SFML and our graphics' structure. SFGUI was a simple and lightweight library so I decided to go with it to save time for the actual UI event system implementation.
Using SFGUI, I created a very simple level editor with object's transformation information is hooked up to the level editor for changing it's values and seeing it in action. That little box of information alone saved a lot of time for level building. As we used it, though, I realized that the design process was still a bit difficult and time-consuming so I came up with a strategy that would require the least amount of time spent on the tools with only the goal of usefulness in mind.
One of my teammates said that adding 3D picking with ray casting is very easy through some online tutorials so he decided to add it to the engine in about a day. Since we had that system implemented, I decided to pass data between that system and the level editor to enable users to be able to "grap" objects and move them around without changing numerical values through the table directly. After this was done, we had a working system for building levels.
While working on the level editor, as a need for the edited files to be saved, I also created a file saving and loading functionality for the engine. After the level editor was up and start running, I realized that any form of data we use would be in JSON format, basically the "save" files we were talking about weren't different from JSON files that defined our levels. I followed that logic and added a file saving/loading options to the level editor. Finally, using this logic, I created our save / load system - which was basically the same thing with saving the level information and states into a JSON file and load it up when needed.
Since my teammates were using the level editor more than I did (due to all the other things needed to be done at the engine) so I used them as my clients to get my requirements and work on them. The next "requirement" was object creation on the level editor. So far, what we had was an editing tool that enabled us to move objects around. Since I had object archetypes and name/tag system built in while implementing JSON serialization on the engine side, I used their names and archetypes to create a list of possible objects to be added to the level. Using this simple feature, we started adding and removing objects from the level which in turn led to an even faster level building.
Towards the end, I rigged other systems to the level editor as well - like physics, some scripting etc... however due to time limitations usefulness of the editor was more important than other development details (like scalability and performance) so I just rigged things that were absolutely important and would worth spending time on. However, even with a number of systems visible to the UI side, the process of building levels and scripting was considerably faster. It used to take us at least a week to get a level set up (without gameplay testing etc - just object placement). With the level editor, I once added an entire level in a day.
![]() Object PickingPicking an object through the level editor. It highlights the selected object and brings out it's properties. |
---|

PARTICLES
GPU-based particles are a known topic for a while now. I didn't know how they work so I asked our graphics developer if I could add something like this to the engine and got my permission to work on his beloved graphics codebase :)
There are many tutorials and documentation that can be found online easily. Ogldev, for example, is one of the famous OpenGL tutorial pages that helped me with many questions in the past. Although I'm assuming this source is enough, I decided to follow through the original document I found in nVidia's website.
The implementation is using a relatively newer technology called Transform Feedback Buffer to transfer data between CPU and GPU, changing values and doing the calculation on GPU side. The idea is to get the particle information frame-by-frame as we process it in the GPU side and draw the new state at each frame. Keeping the loop alive and system fed with new particles using the Geometry Shader and doing the actual drawing and calculation part in the vertex shader is a simple and useful technique to implement a GPU-based particle system.
In addition to the method explained in nVidia's paper, I added a double-buffer structure for switching read-write cycle with a better performance. More information can be found at OglDev tutorials (that's where I got the idea from).
![]() Collectibles without particlesLevel editor doesn't run using the update switch on for particle system (it can be enabled through the settings). This is how the game looks without particles: dull and static. | ![]() Particle SystemGame running with particle systems on. Mind the amount of particles on the screen and how it makes the world more colorful. |
---|
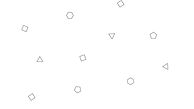
CONCLUSION
As our very first group game project, developing this game taught us many things and improved us any various fields - programming, team management, problem solving etc... I personally enjoyed this project very much, mostly because I'm an architecture-based developer and re-designing a system from scratch is always very fun for me. Also, nearly everything I did in this project was new to me so it was a fresh experience filled with excitement :)
The most challenging part was team mechanics though - especially considering that we were all from different backgrounds and ethnicity, it took us a while to get used to working with each other and finding a system that works. We used Agile methodology for the management, mainly because I had experience using it from my previous job and that took a portion of the burden. I learned that team communication is always the main factor affecting the quality of the final project and there isn't a fixed algorithm that determines what to do always, because no one choice is suitable for everyone so one has to find a way around personalities of team members to create a welcoming environment for all.
An example Settings file of our engine
An example Level file of our engine